Next.js Dynamic Imports for Sitecore
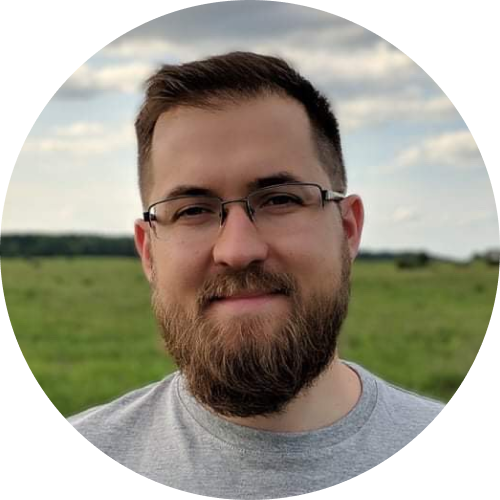

The distinction of Sitecore JSS Next.js websites is that all your pages are rendered by one […path].tsx
file. It is very convenient, content managers control pages and page URLs. Developers take care only about code. However, the peculiarity of Next.js dynamic page URLs is that all JavaScript required for these pages will be added into one big JavaScript chunk.
Let’s look at one example. Imagine, you have a contact page with map component.
You have map
rendering that allows you to add a map to any Sitecore page. But, in your case, you have one or two pages with a map. For example, you will implement your component using Mapbox service.
Your client-side bundle will look similar to this:
And, unfortunately, it will be loaded on all pages. Yes, it will be loaded only once and cached. You will not have any additional loads during the navigation across the site. But that will be the first impression for your client.
Fortunately, you can wrap your heavy component with Dynamic Import.
import dynamic from "next/dynamic";
export const HeavyComponent = dynamic(
() => import("../Heavy-Component").then((mod) => mod.HeavyComponent),
{
loading: () => <p>Loading...</p>,
ssr: false,
}
);
export function DynamicHeavyComponent() {
return <HeavyComponent />;
}
This code will delay the loading of heavy components together with other scripts. If the client doesn’t open the page with the map component, this JavaScript code will never be loaded. You will have much smaller client-side bundle and the first website load will be faster.
Conclusion
Review your code, and know what is inside your client-side bundle. If you have heavy components that are used only on certain pages, wrap them with Dynamic Import and improve your website performance!